How to Use IP Address Lookup API
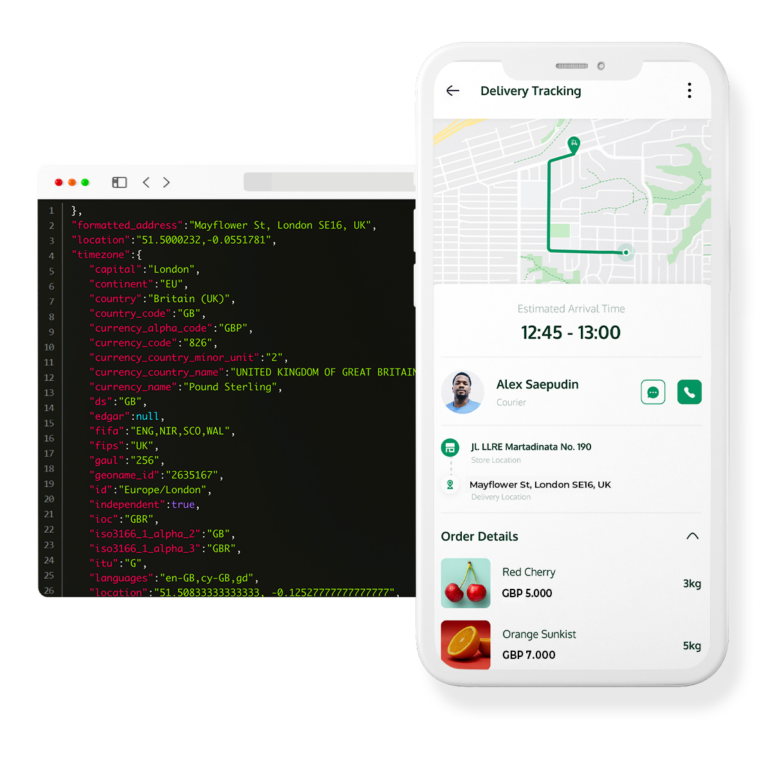
In today’s digital age, getting accurate IP information is crucial for various applications such as fraud detection, geo-targeting, and even analytics. EasyAPI offers two handy endpoints for gathering comprehensive IP information. These endpoints provide data like:
- City and Country: Know where your users are connecting from.
- Timezone: Get the timezone, even down to its specific ID.
- Currency: Identify the currency used in the area.
- Date and Time: Accurate local date and time data.
- Language: Languages spoken in the area.
- State and Postal Code: More granularity on the location.
And much more!
Pre-Requisites
An API key from EasyAPI. If you haven’t obtained this yet, you can refer to our guide on Getting Started Guide.
Step 1: Choose Your Endpoint
EasyAPI offers two endpoints to get IP information:
- For the Current User’s IP:
https://api.easyapi.io/v1.0/ip?api_key=API_KEY
- For a Specific IP Address:
https://api.easyapi.io/v1.0/ip/{ip}?api_key=API_KEY
Decide which one you need for your application.
Step 2: Make an API Request
For Current User's IP
Use the following command in your terminal to make an API call for the current user’s IP.
curl -X GET "https://api.easyapi.io/v1.0/ip?api_key=YOUR_API_KEY"
For a Specific IP Address
Use the following command to get data for a specific IP address, say 82.84.2.2
.
curl -X GET "https://api.easyapi.io/v1.0/ip/82.84.2.2?api_key=YOUR_API_KEY"
Step 3: Examine the Response
The API will return a JSON response similar to this:
{
"class":"address_info",
"properties":{
"datetime":{
"date":"08/23/2023",
"date_time":"08/23/2023 20:39:30",
"date_time_txt":"Wednesday, August 23, 2023 20:39:30",
"date_time_wti":"Wed, 23 Aug 2023 20:39:30 +0100",
"date_time_ymd":"2023-08-23T20:39:30+0100",
"day":"23",
"day_abbr":"Wed",
"day_full":"Wednesday",
"day_wilz":"23",
"hour_12_wilz":"08",
"hour_12_wolz":"8",
"hour_24_wilz":"20",
"hour_24_wolz":"20",
"hour_am_pm":"pm",
"minutes":"39",
"month":"08",
"month_abbr":"Aug",
"month_days":"23",
"month_full":"August",
"month_wilz":"08",
"seconds":"30",
"time":"20:39:30",
"week":"34",
"year":"2023",
"year_abbr":"23"
},
"formatted_address":"Mayflower St, London SE16, UK",
"location":"51.5000232,-0.0551781",
"timezone":{
"capital":"London",
"continent":"EU",
"country":"Britain (UK)",
"country_code":"GB",
"currency_alpha_code":"GBP",
"currency_code":"826",
"currency_country_minor_unit":"2",
"currency_country_name":"UNITED KINGDOM OF GREAT BRITAIN AND NORTHERN IRELAND",
"currency_name":"Pound Sterling",
"ds":"GB",
"edgar":null,
"fifa":"ENG,NIR,SCO,WAL",
"fips":"UK",
"gaul":"256",
"geoname_id":"2635167",
"id":"Europe/London",
"independent":true,
"ioc":"GBR",
"iso3166_1_alpha_2":"GB",
"iso3166_1_alpha_3":"GBR",
"itu":"G",
"languages":"en-GB,cy-GB,gd",
"location":"51.50833333333333, -0.12527777777777777",
"marc":"xxk",
"phone_prefix":"44",
"tld":".uk",
"un_m49_code":"826",
"wmo":"UK"
}
}
}
Understanding the JSON Response Fields
After making a successful API call, you will receive a JSON response. To better understand what each field represents, refer to the following table:
Field Name | Description | Example Values |
---|---|---|
class | Represents the type of data | “ip_info” |
properties.city | The city where the IP is located | “Pescantina” |
properties.country | The country where the IP is located | “Italy” |
properties.country_code | The ISO country code | “IT” |
properties.state | The state or province where the IP is located | “Province of Verona” |
properties.state_code | The state or province code | “VR” |
properties.postal | The postal code of the location | “37026” |
properties.location | Latitude and Longitude coordinates | “45.4802,10.867” |
properties.ip | The IP address | “82.84.2.2” |
properties.datetime | Various formats of the local date and time | See Below for Subfields |
properties.timezone | Various data related to the timezone of the IP | See Below for Subfields |
datetime subfields:
Field Name | Description | Example Values |
---|---|---|
datetime.date | The date | “08/23/2023” |
datetime.time | The time | “21:32:03” |
datetime.year | The year | “2023” |
datetime.month | The month | “08” |
datetime.day | The day | “23” |
timezone subfields:
Field Name | Description | Example Values |
---|---|---|
timezone.id | The timezone ID | “Europe/Rome” |
timezone.capital | The capital city of the country | “Rome” |
timezone.currency_name | The name of the currency used in the country | “Euro” |
timezone.currency_code | The currency code | “978” |
timezone.currency_alpha_code | The ISO alpha code for the currency | “EUR” |
Step 4: Parse the Data
Python Implementation
You can use Python’s requests
library to make the API call and parse the JSON response. Here’s an example:
import requests
import json
response = requests.get("https://api.easyapi.io/v1.0/ip?api_key=YOUR_API_KEY")
data = json.loads(response.text)
city = data["properties"]["city"]
country = data["properties"]["country"]
print(f"City: {city}, Country: {country}")
To run this code, you’ll need to install the requests
library, which can be done via pip:
pip install requests
PHP Implementation
In PHP, you can use curl
to make the API request and json_decode
to parse the response:
Place this code in a PHP file and run it on your web server to get the IP details.
Step 5: Implement in Your Application
After successfully parsing the data, you can use this information in your application for various purposes like analytics, user personalization, or fraud detection.
Both Python and PHP examples demonstrate how easy it is to interact with EasyAPI’s IP information endpoints. Pick the one that fits your needs and integrate it into your application.
Conclusion
Congratulations on successfully navigating through the comprehensive guide on using EasyAPI’s IP Information Endpoints! Understanding the location of your users or clients is vital for a wide array of applications ranging from security measures like fraud detection to customer engagement methods like geo-targeting and analytics.
What's Next?
Getting Started: If you are a newcomer to EasyAPI or need a refresher, make sure to read our Getting Started Guide.
Timezone Detection: Need to fetch time-zone information along with IP info? Check out our Timezone API Guide for another similar endpoint that can enhance your application’s capabilities.
API Documentation: For developers who want an in-depth understanding of all the available functionalities, our API documentation made with redocs is available at https://docs.easyapi.io/.
With this newly acquired knowledge, you are well-equipped to implement IP information into your projects effectively. Happy coding!
Choose the Plan That Fits Your Need
Easy, transparent, and budget-friendly!
Starter
$3/month
- 14 Days FREE
- 5.000 API calls
- VPN detection
- Time zones
- Time zone comparison
- IP Address
- Address info
- Fast support
- Frequent database updates
- Multiple sources
- Commercial use
Commercial
$37/month
- 500.000 API calls per month
- VPN detection
- Time zones
- Time zone comparison
- IP Address
- Address info
- Fast support
- Frequent database updates
- Multiple sources
- Commercial use
Premium
$59/month
- 2 million API calls per month
- VPN detection
- Time zones
- Time zone comparison
- IP Address
- Address info
- Fast support
- Frequent database updates
- Multiple sources
- Commercial use
Business
$139/month
- 6 million API calls per month
- VPN detection
- Time zones
- Time zone comparison
- IP Address
- Address info
- Fast support
- Frequent database updates
- Multiple sources
- Commercial use